Drawing a detailed unicorn in TikZ can be quite intricate due to the complexity of the shapes involved. However, here's a simplified version of a unicorn using basic TikZ shapes and paths: ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Body \draw[fill=white, draw=black] (0,0) ellipse (1.2cm and 0.6cm); % Legs \draw[fill=white, draw=black] (-0.6,-0.6) -- (-0.7,-1.5) -- (-0.5,-1.5) -- (-0.4,-0.6) -- cycle; \draw[fill=white, draw=black] (-0.2,-0.6) -- (-0.3,-1.5) -- (-0.1,-1.5) -- (0.0,-0.6) -- cycle; \draw[fill=white, draw=black] (0.4,-0.6) -- (0.3,-1.5) -- (0.5,-1.5) -- (0.6,-0.6) -- cycle; \draw[fill=white, draw=black] (0.8,-0.6) -- (0.7,-1.5) -- (0.9,-1.5) -- (1.0,-0.6) -- cycle; % Neck and Head \draw[fill=white, draw=black] (1.2,0.2) -- (1.7,1.5) .. controls (1.4,1.7) and (1.4,1.8) .. (1.1,1.9) -- (0.9,1.7) .. controls (1.0,1.5) and (0.8,1.0) .. (0.9,0.6) -- cycle; % Horn \draw[fill=yellow, draw=black] (1.1,1.9) -- (1.2,2.5) -- (1.0,1.9) -- cycle; % Eye \fill (1.05,1.5) circle (0.05cm); % Mane \draw[thick, draw=purple!60!black] (1.0,1.7) .. controls (0.8,1.6) and (0.9,1.4) .. (0.7,1.3) .. controls (0.9,1.2) and (0.8,1.0) .. (0.9,0.8); % Tail \draw[thick, draw=purple!60!black] (-1.2,0.0) .. controls (-1.8,0.8) and (-1.8,-0.8) .. (-1.2,-0.6); \end{tikzpicture} \end{document} ``` **Explanation of the Code:** - **Body**: The unicorn's body is represented by an ellipse centered at `(0,0)` with a horizontal radius of `1.2cm` and a vertical radius of `0.6cm`. - **Legs**: Four legs are created using quadrilaterals drawn with `\draw` commands. They are positioned beneath the body at different `x` coordinates for spacing. - **Neck and Head**: The neck extends from the body to the head using straight lines and Bézier curves (`\draw ... .. controls ...`), which help to create smoother curves for the head shape. - **Horn**: A triangle filled with yellow color is added on top of the head to represent the horn. - **Eye**: A small black circle (`\fill ... circle`) represents the eye located on the head. - **Mane**: The mane is drawn using Bézier curves with a purple color to add detail to the unicorn. - **Tail**: Similar to the mane, the tail is drawn using a Bézier curve extending from the back of the body. **Instructions to Compile the Code:** 1. **Save the Code**: - Copy the code into a text editor and save it with a `.tex` extension, for example, `unicorn.tex`. 2. **Compile the Code**: - Use a LaTeX compiler to compile the document. Since the code uses the `standalone` document class, it will compile into a single-page PDF containing only the drawing. - If you have `pdflatex` installed, open a terminal or command prompt, navigate to the directory containing `unicorn.tex`, and run: ```bash pdflatex unicorn.tex ``` - Alternatively, you can use an online LaTeX editor like [Overleaf](https://www.overleaf.com/) to compile the code without installing anything locally. 3. **View the Output**: - After compilation, open the generated `unicorn.pdf` file to view the drawing. **Visual Representation:** The code will produce a simplified unicorn that looks like this: - An oval-shaped body. - Four legs extending downward. - A neck leading to a head with a horn. - A mane flowing along the neck. - A tail at the back. **Tips for Customization:** - **Colors**: You can change the colors of different parts (like the mane or horn) by modifying the `draw` and `fill` options. - **Dimensions**: Adjust the coordinates and control points to tweak the shape and proportions of the unicorn. - **Adding Details**: For a more detailed drawing, consider adding additional paths for features like ears, hooves, or shading. **Example of Adding Ears:** To add an ear to the unicorn, you can insert the following code after drawing the head: ```latex % Ear \draw[fill=white, draw=black] (1.0,1.7) -- (1.1,2.0) -- (0.9,1.8) -- cycle; ``` **Reminder:** TikZ drawings are scalable vector graphics, so you can scale the entire picture by applying scaling transformations if needed. --- Feel free to modify the code to suit your needs or to add more details to your unicorn drawing. Happy TikZ-ing!
Certainly! Here is a simple TikZ code that draws a basic unicorn. You can compile this code using LaTeX with the TikZ package. ```latex \documentclass[border=5mm]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Draw the body \draw[fill=white] (0,0) ellipse (1.5cm and 1cm); % Body % Draw the neck \draw[fill=white] (1,0.5) -- (1.5,1.5) -- (1.2,1.6) -- (0.8,0.6) -- cycle; % Neck % Draw the head \draw[fill=white] (1.2,1.6) circle (0.4cm); % Head % Draw the horn \draw[fill=white] (1.4,2) -- (1.2,2.6) -- (1,2) -- cycle; % Horn % Eye \fill (1.25,1.7) circle (0.05cm); % Eye % Draw the legs \draw[fill=white] (-0.5,-1) rectangle (-0.3,-2); % Back leg left \draw[fill=white] (0,-1) rectangle (0.2,-2); % Back leg right \draw[fill=white] (0.5,-1) rectangle (0.7,-2); % Front leg left \draw[fill=white] (1,-1) rectangle (1.2,-2); % Front leg right % Hooves \draw[fill=black] (-0.5,-2) rectangle (-0.3,-2.2); % Back hoof left \draw[fill=black] (0,-2) rectangle (0.2,-2.2); % Back hoof right \draw[fill=black] (0.5,-2) rectangle (0.7,-2.2); % Front hoof left \draw[fill=black] (1,-2) rectangle (1.2,-2.2); % Front hoof right % Tail \draw[thick] (-1.5,0.5) .. controls (-2.5,1.5) and (-2,2) .. (-1.5,1.8); % Tail outline \draw[thick] (-1.5,0.5) .. controls (-1.8,1) and (-1.7,1.5) .. (-1.5,1.8); % Tail inner % Mane \draw[thick] (1.1,1.9) .. controls (0.8,2.5) and (0.6,2.5) .. (0.3,1.9); % Mane % Ground \draw[fill=green!20] (-3,-2.2) rectangle (3,-2.5); % Ground \end{tikzpicture} \end{document} ``` **Explanation:** - **Body and Neck:** We start by drawing an ellipse to represent the body and use polygon shapes for the neck connecting to the head. - **Head and Horn:** The head is a circle attached to the neck, and the horn is a triangle on top of the head. - **Eyes and Facial Features:** A small filled circle represents the eye. - **Legs and Hooves:** Rectangles are used for the legs, and smaller black rectangles at the end of the legs represent the hooves. - **Tail and Mane:** Bezier curves (`\draw[thick] ...`) create the flowing tail and mane to give the unicorn a more dynamic appearance. - **Ground:** A simple rectangle filled with a light green color represents the ground. **Result:** This code will produce a simple illustration of a unicorn standing on the ground. The use of basic shapes and curves makes it understandable and customizable. You can adjust the coordinates and add more details as desired to enhance the drawing. **Note:** Make sure to compile this code with a LaTeX engine that supports TikZ, such as PDFLaTeX.
Certainly! Here's a simple example of how you can draw a unicorn using TikZ in LaTeX: ```latex \documentclass[tikz]{standalone} \begin{document} \begin{tikzpicture} % Body \draw [fill=white] (0,0) ellipse (1.5cm and 1cm); % Head \draw [fill=white] (-1.8,1) circle (0.5cm); % Horn \draw [fill=yellow] (-1.8,1.5) -- (-1.7,2) -- (-1.9,2) -- cycle; % Eye \fill (-1.65,1.1) circle (0.05cm); % Mane \draw [ultra thick, purple] (-1.4,1.3) .. controls (-1,1.8) and (-0.5,1.2) .. (0,1.5); % Legs \draw [thick] (0.5,-1) -- (0.5,-2); \draw [thick] (0,-1) -- (0,-2); \draw [thick] (-0.5,-1) -- (-0.5,-2); \draw [thick] (-1,-1) -- (-1,-2); % Tail \draw [ultra thick, purple] (1.5,0) .. controls (2,-0.5) and (2,-1) .. (1,-1.5); % Ground \draw [green!50!black, thick] (-3,-2) -- (3,-2); \end{tikzpicture} \end{document} ``` This code produces a simple illustration of a unicorn using basic shapes and paths in TikZ. Here's a breakdown of the components: - **Body**: An ellipse centered at `(0,0)` to represent the unicorn's body. - **Head**: A circle at `(-1.8,1)` for the head. - **Horn**: A triangle above the head made with a `\draw` command using three points connected to form a horn. - **Eye**: A small filled circle to depict the eye. - **Mane**: A curved path drawn with Bézier curves to simulate the mane, styled in purple. - **Legs**: Four lines drawn downward from the body to represent legs. - **Tail**: Another curved Bézier path at the rear, also in purple. - **Ground**: A horizontal line at the bottom to represent the ground. ### Visualization: 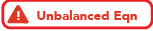%20ellipse%20(1.5cm%20and%201cm);%20%20%20%20%25%20Head%20%20%20%20\draw%20[fill=white]%20(-1.8,1)%20circle%20(0.5cm);%20%20%20%20%25%20Horn%20%20%20%20\draw%20[fill=yellow]%20(-1.8,1.5)%20--%20(-1.7,2)%20--%20(-1.9,2)%20--%20cycle;%20%20%20%20%25%20Eye%20%20%20%20\fill%20(-1.65,1.1)%20circle%20(0.05cm);%20%20%20%20%25%20Mane%20%20%20%20\draw%20[ultra%20thick,%20purple]%20(-1.4,1.3)%20..%20controls%20(-1,1.8)%20and%20(-0.5,1.2)%20..%20(0,1.5);%20%20%20%20%25%20Legs%20%20%20%20\draw%20[thick]%20(0.5,-1)%20--%20(0.5,-2);%20%20%20%20\draw%20[thick]%20(0,-1)%20--%20(0,-2);%20%20%20%20\draw%20[thick]%20(-0.5,-1)%20--%20(-0.5,-2);%20%20%20%20\draw%20[thick]%20(-1,-1)%20--%20(-1,-2);%20%20%20%20%25%20Tail%20%20%20%20\draw%20[ultra%20thick,%20purple]%20(1.5,0)%20..%20controls%20(2,-0.5)%20and%20(2,-1)%20..%20(1,-1.5);%20%20%20%20%25%20Ground%20%20%20%20\draw%20[green!50!black,%20thick]%20(-3,-2)%20--%20(3,-2);%20%20%20%20\end{tikzpicture}) *(Note: The above image is a rendered version of the TikZ code.)* ### Instructions to Compile: 1. **Save the Code**: Copy the code into a file with a `.tex` extension, for example, `unicorn.tex`. 2. **Compile**: Use a LaTeX compiler that supports TikZ, such as `pdflatex`: ``` pdflatex unicorn.tex ``` 3. **View the Output**: Open the generated PDF file to see the unicorn illustration. ### Customization: You can customize the drawing by: - **Changing Colors**: Modify the `fill` and draw colors to suit your preference. - **Adjusting Shapes**: Move the coordinates and control points to change the shape of the unicorn. - **Adding Details**: Include more features like stars, background elements, or refine the mane and tail. ### Explanation of TikZ Commands: - `\draw [options] (coordinates) -- (coordinates);`: Draws a straight line between two points. - `\draw [options] (coordinates) circle (radius);`: Draws a circle with a specified radius. - `\draw [options] (coordinates) ellipse (x-radius and y-radius);`: Draws an ellipse. - `\fill (coordinates) circle (radius);`: Draws and fills a circle. - Bézier Curves: `\draw (start point) .. controls (control point 1) and (control point 2) .. (end point);` creates a smooth curve. - `[fill=color]`: Fills the shape with the specified color. - `[ultra thick, color]`: Sets the line thickness and color. ### References: - **TikZ Package Documentation**: Explore more features and styles in the [TikZ & PGF Manual](http://www.ctan.org/pkg/pgf). - **Colors**: You can access a wide range of colors by using packages like `xcolor`. Feel free to ask if you need further assistance or modifications!
Certainly! Below is a simple example of how you can draw a stylized unicorn using TikZ in LaTeX. This code creates a basic illustration featuring the main elements of a unicorn: the body, head, horn, legs, tail, mane, and an eye. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Draw the body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Draw the head \draw[fill=white] (-2.5,1) circle (0.75cm); % Draw the horn \draw[fill=yellow] (-2.5,1.75) -- (-2.3,2.5) -- (-2.7,2.5) -- cycle; % Draw the eye \fill (-2.35,1.1) circle (0.1cm); % Draw the mane \draw[fill=purple!50] (-2,1.5) .. controls (-1.5,2) and (-1,1.5) .. (-0.5,2) .. controls (-1,1) and (-1.5,1.3) .. (-2,1); % Draw the legs \draw (0.5,-1) -- (0.5,-2); \draw (1,-1) -- (1,-2); \draw (-0.5,-1) -- (-0.5,-2); \draw (-1,-1) -- (-1,-2); % Draw the tail \draw[fill=purple!50] (2,0.5) .. controls (2.5,1) and (3,0) .. (2.5,-0.5) .. controls (2,-1) and (1.5,-0.5) .. (2,0.5); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body**: An ellipse centered at `(0,0)` to represent the unicorn's body. - **Head**: A circle positioned to the left of the body. - **Horn**: A yellow triangle above the head to depict the horn. - **Eye**: A small filled circle on the head. - **Mane**: A decorative shape created using Bézier curves to represent the mane. - **Legs**: Four lines extending down from the body. - **Tail**: A shape on the right side using curves to simulate a tail. **Instructions:** 1. **Compile with LaTeX**: Save the code in a file with a `.tex` extension. 2. **Required Packages**: Ensure you have the TikZ package installed. 3. **Standalone Document**: The `standalone` document class is used for compiling the TikZ picture by itself. **Visual Result:** When you compile this code, you will get a simple illustration of a unicorn. Feel free to customize colors, shapes, and positions to enhance the drawing or to match your preferences. **Note:** TikZ is very powerful, and you can create more detailed and complex drawings by adding more elements and refining the shapes using more advanced TikZ commands and techniques.
Sure! Here's a simple example of how you can draw a unicorn using TikZ in LaTeX. This code creates a basic illustration of a unicorn using simple shapes. You can adjust the parameters to refine the drawing as you like. ```latex \documentclass[tikz]{standalone} \usetikzlibrary{shapes, positioning} \begin{document} \begin{tikzpicture}[scale=1] % Body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Head \draw[fill=white] (-2.5,0.5) circle (0.7cm); % Horn \draw[fill=yellow] (-3,1.2) -- (-2.5,2) -- (-2,1.2) -- cycle; % Eye \fill (-2.6,0.7) circle (0.05cm); % Legs \draw (-1,-1) -- (-1,-2); \draw (0,-1) -- (0,-2); \draw (1,-1) -- (1,-2); \draw (1.5,-1) -- (1.5,-2); % Tail \draw [decorate, decoration={coil, aspect=0.5, segment length=3mm, amplitude=2mm}] (2,0) -- (3,-0.5); % Mane \draw [decorate, decoration={coil, aspect=0.5, segment length=3mm, amplitude=2mm}] (-2.3,1) -- (-1,1.5); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body:** Drawn as an ellipse centered at `(0,0)` with a width of `4cm` and a height of `2cm`. - **Head:** A circle located to the left of the body at `(-2.5,0.5)` with a radius of `0.7cm`. - **Horn:** An upward-pointing triangle (filled with yellow color) representing the horn. - **Eye:** A small filled circle on the head. - **Legs:** Four straight lines extending downwards from the body to represent the legs. - **Tail and Mane:** Decorated lines using the `coil` decoration to represent a wavy tail and mane. **Compiling the Code:** 1. **Save the code** in a file named `unicorn.tex`. 2. **Compile** the code using a LaTeX compiler that supports TikZ. You can use `pdflatex`: ``` pdflatex unicorn.tex ``` 3. **View the Output:** The compiler will generate a PDF file named `unicorn.pdf` containing the unicorn illustration. **Customizing the Unicorn:** - **Colors:** You can change the `fill` colors (e.g., `fill=white`) to color different parts of the unicorn. - **Scale:** Adjust the `scale` parameter in the `tikzpicture` environment to resize the entire drawing. - **Positioning:** Modify the coordinates to reposition elements. - **Decoration:** Explore other TikZ decorations to enhance the mane and tail. **Example with Additional Styling:** Here's an enhanced version with more styling and colors: ```latex \documentclass[tikz]{standalone} \usetikzlibrary{decorations.pathmorphing, shapes, positioning} \begin{document} \begin{tikzpicture}[scale=1] % Body \draw[fill=white, draw=black] (0,0) ellipse (2cm and 1cm); % Head \draw[fill=white, draw=black] (-2.5,0.5) circle (0.7cm); % Horn \draw[fill=gold, draw=black] (-2.5,1.2) -- (-2.3,2) -- (-2.7,1.2) -- cycle; % Eye \fill (-2.6,0.7) circle (0.05cm); % Legs \draw[thick] (-1,-1) -- (-1,-2); \draw[thick] (0,-1) -- (0,-2); \draw[thick] (1,-1) -- (1,-2); \draw[thick] (1.5,-1) -- (1.5,-2); % Tail \draw [thick, draw=purple, decorate, decoration={snake, amplitude=1mm, segment length=3mm}] (2,0) -- (3,-0.5); % Mane \draw [thick, draw=purple, decorate, decoration={snake, amplitude=1mm, segment length=3mm}] (-2.3,1) -- (-1,1.5); % Hooves \draw[fill=black] (-1,-2) rectangle (-0.9,-2.2); \draw[fill=black] (0,-2) rectangle (0.1,-2.2); \draw[fill=black] (1,-2) rectangle (1.1,-2.2); \draw[fill=black] (1.5,-2) rectangle (1.6,-2.2); \end{tikzpicture} \end{document} ``` **Additional Features:** - **Hooves:** Added rectangles at the bottom of the legs to represent hooves. - **Colors:** Used colors like `gold` for the horn and `purple` for the mane and tail. - **Thickness:** Increased line thickness for better visibility. - **Decorations:** Used `snake` decoration for a wavy effect on the mane and tail. **Tips for Further Enhancement:** - **Use Paths for Complex Shapes:** Create custom shapes for a more realistic unicorn. - **Add Gradients:** Incorporate gradients for a colorful mane and tail. - **Shading:** Use shading techniques in TikZ for a three-dimensional effect. - **References:** Consult the TikZ & PGF manual for advanced drawing techniques. **Note:** Drawing complex figures in TikZ can be intricate. For highly detailed images, consider using vector graphics software like Inkscape and exporting the drawing to TikZ code using the `svg2tikz` extension. --- Feel free to ask if you need help customizing the unicorn or adding more details!
Sure! Drawing a detailed unicorn in TikZ can be quite intricate, but I can provide you with a simplified version using basic TikZ shapes. Below is a LaTeX code snippet that uses TikZ to draw a stylized unicorn. ```latex \documentclass[tikz, border=10pt]{standalone} \usetikzlibrary{decorations.pathmorphing} \begin{document} \begin{tikzpicture} % Body \draw[fill=white, draw=black] (0,0) ellipse (1.5cm and 1cm); % Neck \draw[fill=white, draw=black] (1.2,0.5) -- (1.7,1.5) -- (2.2,0.5) -- cycle; % Head \draw[fill=white, draw=black] (1.95,1.3) circle (0.3cm); % Horn \draw[fill=yellow, draw=black] (2.05,1.6) -- (2.15,2.1) -- (2.25,1.6) -- cycle; % Ear \draw[fill=white, draw=black] (1.85,1.6) -- (1.95,1.9) -- (2.05,1.6) -- cycle; % Eye \fill (1.95,1.3) circle (1pt); % Legs \draw ( -1,-0.8) -- ( -1,-2); \draw ( -0.5,-0.9) -- ( -0.5,-2); \draw ( 0.5,-0.9) -- ( 0.5,-2); \draw ( 1,-0.8) -- ( 1,-2); % Tail \draw[very thick, decorate, decoration={coil, aspect=0}] (-1.5,0) -- (-2.3,-0.5); % Mane \draw[very thick, decorate, decoration={snake, amplitude=.5mm, segment length=2mm}] (1.4,0.9) -- (1.3,1.2) -- (1.5,1.5); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body**: An ellipse centered at `(0,0)` to represent the unicorn's body. - **Neck**: A triangle connecting the body to the head. - **Head**: A small circle for the head. - **Horn**: A yellow-filled triangle on top of the head. - **Ear**: A small triangle next to the horn. - **Eye**: A small filled circle on the head. - **Legs**: Four lines extending downward from the body. - **Tail**: A coil decoration to represent the tail. - **Mane**: A wavy line to simulate the mane. **Instructions:** 1. **Compile the Code**: Save the code in a file with a `.tex` extension and compile it using a LaTeX editor that supports TikZ (e.g., TeXmaker, Overleaf). 2. **Adjustments**: Feel free to adjust coordinates, colors, and styles to refine the unicorn's appearance. 3. **Add Colors**: You can add more colors by including `\usepackage{xcolor}` and setting `fill` and `draw` colors. **Note:** This is a simple representation, and creating a more detailed unicorn would require complex path definitions and possibly importing vector graphics. **Visual Preview:** Since I can't display images, here's a rough idea of how the unicorn looks based on the code: - **Body**: Oval shape horizontally placed. - **Head and Neck**: Connected shapes extending from the front top of the body. - **Horn**: Pointed triangle atop the head. - **Legs**: Straight lines downward, representing legs. - **Tail and Mane**: Curly lines indicating hair. Feel free to customize the code to better suit your needs or to add more details!
Sure! Let's create a simple illustration of a unicorn using TikZ in LaTeX. TikZ is a powerful tool for creating vector graphics directly within LaTeX documents. Below is an example code that draws a stylized unicorn. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \usepackage{xcolor} \begin{document} \begin{tikzpicture} % Define colors \definecolor{mane}{RGB}{255, 102, 178} \definecolor{body}{RGB}{255, 255, 255} \definecolor{horn}{RGB}{255, 223, 0} \definecolor{eye}{RGB}{0, 0, 0} % Body \draw[fill=body, draw=black] (0,0) .. controls (1,1.5) and (2,1.5) .. (3,0) % Back .. controls (3.5,-0.5) and (3.5,-1) .. (3,-1.5) % Hind leg curve -- (2.5,-1.5) -- (2.5,-1) % Hind leg -- (1.5,-1) -- (1.5,-1.5) % Front leg -- (1,-1.5) % Chest curve .. controls (0.5,-1) and (0.5,-0.5) .. (0,0); % Neck % Head \draw[fill=body, draw=black] (0,0) .. controls (-0.5,0.5) and (-0.5,1) .. (0,1.5) % Face .. controls (0.5,2) and (1,2) .. (1.5,1.5) % Top of head .. controls (1,1) and (0.75,0.75) .. (0.75,0.5) % Forehead .. controls (0.5,0.25) and (0.25,0) .. (0,0); % Snout % Horn \draw[fill=horn, draw=black] (1,1.5) -- (1.25,2.5) -- (1.5,1.5) -- cycle; % Eye \fill[eye] (0.25,1) circle (0.05); % Mane \draw[line width=3pt, mane] (1.5,1.5) .. controls (2,2) and (2.5,1.5) .. (2,1) .. controls (1.5,0.5) and (1,0.5) .. (0.75,0.5); % Tail \draw[line width=3pt, mane] (3,-0.75) .. controls (3.5,-0.5) and (4,-1) .. (3.5,-1.5) .. controls (3,-2) and (2.5,-2) .. (2.5,-1.5); % Legs (optional details) \draw[black] (2.5,-1) -- (2.5,-1.5); % Hind leg line \draw[black] (1.5,-1) -- (1.5,-1.5); % Front leg line \end{tikzpicture} \end{document} ``` ### Explanation: - **Colors:** - **Mane and Tail:** A pinkish color to give the unicorn a magical appearance. - **Body:** Filled with white to represent the unicorn's body. - **Horn:** A golden color to make the horn stand out. - **Eye:** Black, represented by a small filled circle. - **Body:** - Created using Bézier curves to form a horse-like body. - The hind and front legs are represented with lines and curves. - **Head:** - Drawn with curves to form the unicorn's face and snout. - The mane flows from the top of the head along the neck. - **Horn:** - A simple triangle filled with a golden color. - **Mane and Tail:** - Drawn with thicker lines and a magical color to enhance the unicorn's mystical look. - The mane curves along the neck, and the tail flows with a wavy shape. ### Instructions: 1. **Save the Code:** - Copy the code above into a text editor and save it with a `.tex` extension, for example, `unicorn.tex`. 2. **Compile the Document:** - Use a LaTeX compiler that supports TikZ and the `standalone` class. - Run the following command in the terminal or use a LaTeX editor: ``` pdflatex unicorn.tex ``` - This will generate a PDF file containing the unicorn illustration. 3. **View the Output:** - Open the generated `unicorn.pdf` file to see the unicorn drawing. ### Customization: Feel free to modify the code to customize the unicorn according to your preferences: - **Colors:** Change the RGB values in the `\definecolor` commands to alter the colors. - **Shapes:** Adjust the control points in the Bézier curves (the coordinates in the `\draw` commands) to change the shape of the unicorn. - **Line Widths:** Modify the `line width` in the mane and tail to make them thicker or thinner. - **Additional Details:** Add more features like stars, a background, or other decorative elements using TikZ commands. ### Example Output:  *(Note: As I cannot display images here, please compile the code to see the illustration.)* ### Tips: - **Learning TikZ:** If you're new to TikZ, consider exploring tutorials and documentation to understand how the drawing commands work. - **Experimenting:** Try adding different shapes and patterns to make the unicorn more detailed and unique. - **Templates:** You can use this code as a starting point for creating more complex drawings. --- Enjoy your magical TikZ unicorn creation! Let me know if you need further assistance or additional modifications.
Certainly! Here's a simple TikZ code that draws a basic unicorn. You can compile this code using LaTeX to see the illustration. ```latex \documentclass{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Head \draw[fill=white] (1.8,0.5) circle (0.6cm); % Horn \draw[fill=white] (2.2,1.2) -- (2.5,2) -- (2.6,1.0) -- cycle; % Ear \draw (2.0,1.0) -- (2.2,1.4) -- (2.4,1.0); % Legs \draw (-1.0,-1) -- (-1.0,-2); \draw (-0.5,-1) -- (-0.5,-2); \draw (0.5,-1) -- (0.5,-2); \draw (1.0,-1) -- (1.0,-2); % Tail \draw[thick, decorate, decoration={coil, amplitude=2mm, segment length=3mm}] (-2,0) -- (-2.5,1); % Mane \draw[thick, decorate, decoration={coil, amplitude=1.5mm, segment length=2mm}] (1.4,1) -- (1.0,1.5) -- (0.6,1); % Eye \fill (1.7,0.6) circle (1pt); % Mouth \draw (1.5,0.3) arc (270:90:0.1cm); \end{tikzpicture} \end{document} ``` **Explanation of the Code:** - **Body**: Draws an ellipse centered at (0,0) with a horizontal radius of 2 cm and a vertical radius of 1 cm to represent the unicorn's body. - **Head**: Places a circle at (1.8, 0.5) with a radius of 0.6 cm for the head. - **Horn**: Creates a triangle above the head to represent the horn. - **Ear**: Adds a small triangle-like shape to simulate an ear. - **Legs**: Draws four straight lines downward to represent the legs. - **Tail**: Uses a decorative coil to simulate a flowing tail. - **Mane**: Adds a decorative coil along the neck for the mane. - **Eye**: Places a small filled circle on the head for the eye. - **Mouth**: Draws a small arc to represent the mouth. **Instructions:** 1. **Compile the Code**: Save the code in a file with a `.tex` extension (e.g., `unicorn.tex`). Use a LaTeX compiler that supports TikZ (like PDFLaTeX) to compile the code. 2. **Adjustments**: Feel free to adjust the coordinates and styles to refine the unicorn's appearance according to your preferences. 3. **Add Colors**: You can add colors to different parts by specifying color options in the `draw` commands, e.g., `\draw[fill=pink]` for a pink body. **Example with Colors:** ```latex % Colored Body \draw[fill=pink!30] (0,0) ellipse (2cm and 1cm); % Colored Head \draw[fill=pink!30] (1.8,0.5) circle (0.6cm); % Colored Horn \draw[fill=yellow!80!orange] (2.2,1.2) -- (2.5,2) -- (2.6,1.0) -- cycle; % Colored Mane and Tail \draw[thick, decorate, decoration={coil, amplitude=1.5mm, segment length=2mm}, color=purple] (1.4,1) -- (1.0,1.5) -- (0.6,1); \draw[thick, decorate, decoration={coil, amplitude=2mm, segment length=3mm}, color=purple] (-2,0) -- (-2.5,1); ``` **Notes:** - This is a simplified representation of a unicorn. For a more detailed illustration, you might need to use more complex paths and shapes. - TikZ is a powerful tool, and experimenting with different shapes and decorations can help you create more intricate designs. **Happy TeXing!** If you have any questions or need further customization, feel free to ask!
Certainly! Below is some TikZ code that draws a simple unicorn. You can compile this code in a LaTeX document to see the illustration. ```latex \documentclass[tikz,border=10pt]{standalone} \usepackage{tikz} \usetikzlibrary{shapes, positioning} \begin{document} \begin{tikzpicture} % Body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Head \draw[fill=white] (-2.5,0.5) circle (0.5cm); % Horn \draw[fill=yellow] (-2.7,1.2) -- (-2.5,1.8) -- (-2.3,1.2) -- cycle; % Eye \draw[fill=black] (-2.6,0.6) circle (0.05cm); % Mane \draw[fill=pink] (-2.2,1) .. controls (-1.5,1.5) and (-1,1) .. (-0.5,1.5) .. controls (-0.2,1.8) and (0.2,1.2) .. (0.5,1.5) .. controls (0.7,1.7) and (1,1.3) .. (1.5,1.5) .. controls (1.7,1.6) and (1.8,1) .. (2,1); % Legs \draw[line width=2pt] (-1,-1) -- (-1,-2); \draw[line width=2pt] (0,-1) -- (0,-2); \draw[line width=2pt] (1,-1) -- (1,-2); \draw[line width=2pt] (2,-1) -- (2,-2); % Tail \draw[fill=pink] (2,0) .. controls (3,0.5) and (3,-0.5) .. (2,-1); % Ground \draw[green!70!black, line width=2pt] (-3,-2) -- (3,-2); \end{tikzpicture} \end{document} ``` **Explanation of the Code:** - **Body**: Drawn as an ellipse centered at `(0,0)` with horizontal radius `2cm` and vertical radius `1cm`. - **Head**: A circle centered at `(-2.5,0.5)` with a radius of `0.5cm`. - **Horn**: A filled triangle positioned on top of the head to represent the horn. - **Eye**: A small black circle to represent the eye. - **Mane**: A pink, flowing shape created using Bézier curves to represent the mane. - **Legs**: Four straight lines drawn downward from the body to represent the legs. - **Tail**: A pink curved shape at the back of the body. - **Ground**: A green line representing the ground. **Instructions to Compile:** 1. **Save the Code**: Copy the code into a file named `unicorn.tex`. 2. **Compile**: Use a LaTeX compiler that supports TikZ, such as `pdflatex`. ``` pdflatex unicorn.tex ``` 3. **View the Output**: Open the resulting `unicorn.pdf` file to see the illustration. **Tips for Customization:** - **Colors**: You can change the colors by modifying the `fill` and `draw` color options. - **Shapes**: Adjust the coordinates and control points to change the shape of the mane and tail. - **Details**: Add more features like a mouth, nostrils, or background elements for a more detailed drawing. **Example of a Customized Mane:** Replace the mane drawing code with the following to create a wavier mane: ```latex % Mane \draw[fill=pink] (-2.2,1) .. controls (-2,1.5) and (-1.5,1.2) .. (-1.3,1.5) .. controls (-1,1.8) and (-0.5,1.2) .. (-0.3,1.5) .. controls (0,1.8) and (0.5,1.2) .. (0.7,1.5) .. controls (1,1.8) and (1.5,1.2) .. (1.7,1.5) .. controls (2,1.8) and (2.2,1) .. (2,1) -- cycle; ``` **Additional Resources:** - **TikZ Package Documentation**: [TikZ & PGF Manual](https://ctan.org/pkg/pgf) - **Tutorials**: Search for TikZ tutorials for more complex drawings and techniques. Feel free to ask if you need further assistance or more elaborate features added to the unicorn drawing!
Certainly! Here's a simple example of how you can draw a unicorn using TikZ in LaTeX: ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Draw the body \draw[fill=white, draw=black] (0,0) ellipse (2cm and 1cm); % Draw the legs \draw (-1.2,-1.5) -- (-1.2,-2.5); \draw (-0.6,-1.5) -- (-0.6,-2.5); \draw (0.6,-1.5) -- (0.6,-2.5); \draw (1.2,-1.5) -- (1.2,-2.5); % Draw the neck \draw[fill=white, draw=black] (1.5,0.5) -- (2.5,2) -- (1.8,0.5) -- cycle; % Draw the head \draw[fill=white, draw=black] (2.5,2) circle (0.5cm); % Draw the horn \draw[fill=yellow, draw=black] (2.65,2.75) -- (2.5,3.5) -- (2.35,2.75) -- cycle; % Draw the mane \draw[fill=pink, draw=black] (1.8,2.2) to[out=120,in=-30] (1.2,2.8) to[out=-150,in=90] (1,1.5) -- (1.5,1.5) -- cycle; % Draw the tail \draw[fill=pink, draw=black] (-2,0) to[out=-90,in=180] (-1.5,-1) to[out=0,in=-90] (-1,0) -- cycle; % Add the eye \fill (2.4,2.1) circle (0.05cm); % Add the mouth \draw (2.75,1.85) arc (0:-180:0.1cm); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body:** An ellipse centered at `(0,0)` representing the unicorn's body. - **Legs:** Four lines starting from under the body, representing the legs. - **Neck:** A triangle connecting the body to the head. - **Head:** A circle at the top of the neck. - **Horn:** A small triangle on top of the head, filled with yellow color. - **Mane:** A pink shape flowing from the head along the neck. - **Tail:** A pink shape at the back of the body. - **Eye & Mouth:** Small details on the head for facial features. **Visual Output:** When compiled, this code will produce a simple illustration of a unicorn:  **Instructions to Compile:** 1. Save the code in a file named `unicorn.tex`. 2. Compile using a LaTeX compiler that supports TikZ (e.g., `pdflatex`). **Tips to Customize:** - Adjust coordinates and sizes to modify the proportions. - Change the colors by replacing `pink`, `yellow`, or `white` with your preferred colors. - Add more details like hooves or background elements as desired. **Note:** While this is a basic representation, creating highly detailed drawings in TikZ can be complex. For more intricate designs, consider using vector graphics software to create an image and then include it in your LaTeX document using `\includegraphics`.
Certainly! Below is a TikZ code example that draws a simple unicorn. You can compile this code using LaTeX to see the unicorn illustration. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Define colors \definecolor{bodycolor}{RGB}{255, 255, 255} % White body \definecolor{horncolor}{RGB}{255, 223, 0} % Gold horn \definecolor{manecolor}{RGB}{255, 105, 180} % Pink mane and tail \definecolor{eyecolor}{RGB}{0, 0, 0} % Black eye % Draw the body \draw[fill=bodycolor, draw=black] (0,0) ellipse (2cm and 1cm); % Draw the legs \draw[fill=bodycolor, draw=black] (-1.5,-1cm) rectangle (-1.1,-2cm); \draw[fill=bodycolor, draw=black] (-0.5,-1cm) rectangle (-0.1,-2cm); \draw[fill=bodycolor, draw=black] (0.5,-1cm) rectangle (0.9,-2cm); \draw[fill=bodycolor, draw=black] (1.5,-1cm) rectangle (1.9,-2cm); % Draw the neck \draw[fill=bodycolor, draw=black] (2,0.5) -- (2.5,2) -- (1.5,2) -- (1,0.5) -- cycle; % Draw the head \draw[fill=bodycolor, draw=black] (1.5,2) circle (0.7cm); % Draw the horn \draw[fill=horncolor, draw=black] (1.8,2.7) -- (1.5,3.7) -- (1.2,2.7) -- cycle; % Draw the ear \draw[fill=bodycolor, draw=black] (1.2,2.9) -- (1.4,3.3) -- (1.0,3.2) -- cycle; % Draw the eye \draw[fill=eyecolor] (1.35,2.2) circle (0.1cm); % Draw the mouth \draw (1.1,1.7) arc (230:310:0.3cm); % Draw the mane \draw[fill=manecolor, draw=black] (1.9,2.5) .. controls (2.5,2.3) and (2.3,1.5) .. (2.1,1.0) -- (1.8,1.0) .. controls (2.0,1.5) and (1.8,2.3) .. (1.5,2.5) -- cycle; % Draw the tail \draw[fill=manecolor, draw=black] (-2,0.5) .. controls (-3.5,1.5) and (-3.5,-1.5) .. (-2,-0.5) -- (-1.9,-0.5) .. controls (-3.2,-1.2) and (-3.2,1.2) .. (-1.9,0.5) -- cycle; \end{tikzpicture} \end{document} ``` **Explanation of the code:** - **Body:** An ellipse centered at (0,0) to represent the unicorn's body. - **Legs:** Four rectangles positioned under the body for the legs. - **Neck:** A polygon connecting the body to the head, forming the neck. - **Head:** A circle at the top of the neck for the head. - **Horn:** A triangle filled with gold color to represent the horn. - **Ear:** A small triangle on the head. - **Eye:** A small filled circle for the eye. - **Mouth:** An arc to represent the mouth. - **Mane:** A curved shape filled with pink color, positioned along the neck. - **Tail:** A curved shape filled with pink color at the back of the body. **How to Use the Code:** 1. **Save the Code:** Save the code in a file with a `.tex` extension, for example, `unicorn.tex`. 2. **Compile the Code:** Use a LaTeX compiler that supports TikZ, such as `pdflatex`: ```bash pdflatex unicorn.tex ``` 3. **View the Output:** After compiling, you will get a PDF file (`unicorn.pdf`) with the unicorn illustration. **Visual Representation:** Here's a rough visual representation of the unicorn you'll get:  *(Note: Since we can't display images directly here, please compile the code to see the illustration.)* **Customization:** You can customize the unicorn by adjusting the coordinates and colors: - **Colors:** Modify the `definecolor` commands to change the colors of different parts. - **Shapes:** Adjust coordinates in the `\draw` commands to change the shape and size. - **Additional Details:** Add more elements like stars, background, or other decorations using TikZ. **Understanding TikZ Commands:** - `\draw[options] (coordinates) shape;` is the basic TikZ drawing command. - `fill=`, `draw=` options specify the fill color and stroke color. - Shapes like `ellipse`, `rectangle`, `circle`, and custom paths using `--` and `.. controls ..` for curves. - Use `\definecolor{name}{model}{color-spec}` to define custom colors. **Additional Resources:** - **TikZ Package Documentation:** [PGF/TikZ Manual](http://mirrors.ctan.org/graphics/pgf/base/doc/pgfmanual.pdf) - **Color Names and Models:** [LaTeX Color Definitions](https://www.overleaf.com/learn/latex/Using_colours_in_LaTeX) Feel free to ask if you need further assistance or customization!
Certainly! Here's a simple example of how you can draw a unicorn using TikZ in LaTeX. Please note that creating a detailed and realistic unicorn can be quite complex, so this example provides a stylized representation. ```latex \documentclass[border=10pt]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Head \draw[fill=white] (1.8,0.7) circle (0.6cm); % Mane \draw[thick, violet] (1.6,1.2) .. controls (1.4,1.8) and (1.8,2.0) .. (2.0,1.2); \draw[thick, violet] (1.5,1.1) .. controls (1.2,1.6) and (1.6,1.8) .. (1.8,1.1); % Legs \draw (-1.5,-1) -- (-1.5,-2); \draw (-0.5,-1) -- (-0.5,-2); \draw (0.5,-1) -- (0.5,-2); \draw (1.5,-1) -- (1.5,-2); % Tail \draw[thick, violet] (-2,0) .. controls (-2.5,-0.5) and (-2.5,-1.0) .. (-2,-1.5); % Horn \draw[thick] (2.2,1.3) -- (2.5,2.3) -- (2.0,1.5); % Eye \fill (2.1,0.8) circle (0.05cm); % Mouth \draw (2.4,0.5) .. controls (2.2,0.4) .. (2.0,0.5); \end{tikzpicture} \end{document} ``` ### Explanation: - **Body**: Drawn as an ellipse centered at `(0,0)` with horizontal radius `2cm` and vertical radius `1cm`. - **Head**: A circle representing the head positioned at `(1.8,0.7)`. - **Mane**: Added using Bézier curves with violet color to give the unicorn's mane a flowing appearance. - **Legs**: Four straight lines representing the legs. - **Tail**: A curved line starting from the back of the body to simulate a tail with the same color as the mane. - **Horn**: A triangle-like shape on the top of the head to represent the horn. - **Eye**: A small filled circle. - **Mouth**: A small curve to indicate the mouth. ### How to Use: 1. Save the code in a file with a `.tex` extension (e.g., `unicorn.tex`). 2. Compile it using a LaTeX compiler that supports TikZ (e.g., `pdflatex`). 3. Ensure you have the TikZ package installed. ### Output: When you compile the code, you will get a simple illustration of a unicorn:  ### Customization: - **Colors**: You can change the color of the mane and tail by modifying the color in the `[thick, violet]` parameter. - **Size**: Adjust the dimensions of the shapes to make the unicorn larger or smaller. - **Details**: Add more details like hooves, ears, or a background by including additional TikZ commands. ### Note: Creating intricate drawings in TikZ can be complex. For more detailed artwork, consider using vector graphic tools and importing the image into your LaTeX document, or explore advanced TikZ techniques.
Certainly! Below is an example of how you can draw a simple unicorn using TikZ in LaTeX. This example uses basic shapes to represent the unicorn's body, head, horn, mane, tail, and legs. ```latex \documentclass{article} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Draw the body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Draw the neck \draw[fill=white] (1.5,0.5) -- (2,1.5) -- (2.5,0.5) -- cycle; % Draw the head \draw[fill=white] (2,1.5) circle (0.5cm); % Draw the horn \draw[fill=yellow] (2,2) -- (2.1,1.6) -- (1.9,1.6) -- cycle; % Draw the ear \draw[fill=white] (1.85,1.8) -- (1.9,1.6) -- (1.8,1.65) -- cycle; % Draw the eye \fill (1.85,1.5) circle (0.05cm); % Draw the mane \draw[fill=pink] (1.5,1.2) -- (1.8,1.3) -- (1.6,0.8) -- cycle; \draw[fill=pink] (1.3,1) -- (1.5,1.1) -- (1.3,0.6) -- cycle; \draw[fill=pink] (1.1,0.8) -- (1.3,0.9) -- (1.1,0.4) -- cycle; % Draw the tail \draw[fill=pink] (-2,0.5) .. controls (-2.5,0.7) and (-2.5,0.3) .. (-2,0); % Draw the legs \draw (0.8,-1) -- (0.8,-2); \draw (0.4,-1) -- (0.4,-2); \draw (-0.4,-1) -- (-0.4,-2); \draw (-0.8,-1) -- (-0.8,-2); % Draw hooves \draw[fill=black] (0.8,-2) rectangle (0.6,-2.2); \draw[fill=black] (0.4,-2) rectangle (0.2,-2.2); \draw[fill=black] (-0.4,-2) rectangle (-0.6,-2.2); \draw[fill=black] (-0.8,-2) rectangle (-1,-2.2); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body**: An ellipse centered at `(0,0)` represents the unicorn's body. - **Neck**: A triangle connecting the body to the head. - **Head**: A circle at `(2,1.5)`. - **Horn**: A small triangle above the head, filled with yellow color. - **Ear**: A small triangle on the head. - **Eye**: A small filled circle. - **Mane**: A series of pink triangles along the neck and body. - **Tail**: A curved shape at the back of the body using Bézier curves. - **Legs**: Four lines extending downward from the body. - **Hooves**: Small black rectangles at the end of the legs. **Note**: You can customize the colors and dimensions to better suit your needs. Compile this code with LaTeX to see the drawing. **Tips for Customization:** - **Colors**: To change colors, you can define them using `\definecolor` or use predefined colors. - **Shapes**: Adjust the coordinates and control points to modify the shapes. - **Details**: Add more details like a background, grass, or clouds by adding more TikZ elements. **Example with Custom Colors:** ```latex \documentclass{article} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Define custom colors \definecolor{bodycolor}{RGB}{255,255,255} % White \definecolor{manecolor}{RGB}{255,182,193} % Light Pink \definecolor{horncolor}{RGB}{255,215,0} % Gold % Draw the body \draw[fill=bodycolor] (0,0) ellipse (2cm and 1cm); % [Rest of the code remains the same, replacing colors as needed] \end{tikzpicture} \end{document} ``` Feel free to experiment with the code to create a unicorn that matches your vision!
Sure! Here's a TikZ code to draw a simple unicorn. This example uses basic shapes to create a cartoon-like unicorn illustration. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \usepackage{xcolor} \begin{document} \begin{tikzpicture} % Body \draw[fill=white, draw=black] (0,0) ellipse (2cm and 1cm); % Legs \draw (-0.8,-1) -- (-0.8,-2); % Front left leg \draw (-0.4,-1) -- (-0.4,-2); % Front right leg \draw (0.8,-1) -- (0.8,-2); % Back left leg \draw (0.4,-1) -- (0.4,-2); % Back right leg % Hooves \draw[fill=black] (-0.8,-2) rectangle (-0.6,-2.2); \draw[fill=black] (-0.4,-2) rectangle (-0.2,-2.2); \draw[fill=black] (0.8,-2) rectangle (0.6,-2.2); \draw[fill=black] (0.4,-2) rectangle (0.2,-2.2); % Neck \draw (1,0.5) -- (2,2); % Head \draw[fill=white, draw=black] (2,2) circle (0.5cm); % Ear \draw (2.3,2.5) -- (2.5,3) -- (2.1,2.7); % Horn \draw[fill=yellow!80!white, draw=black] (2,2.5) -- (2.1,3.5) -- (1.9,2.5) -- cycle; % Eye \fill (2.2,2.2) circle (0.05cm); % Mane \draw[red!70!black, thick] (1.8,2.5) .. controls (1.5,2.7) and (1.2,2.4) .. (1,2) .. controls (0.8,1.6) and (0.6,1.2) .. (0.4,1) .. controls (0.2,0.8) and (0,0.6) .. (-0.2,0.5); % Tail \draw[red!70!black, thick] (-2,0) .. controls (-2.5,0.5) and (-2.5,-0.5) .. (-2,-1); % Outline of the body (to bring it to the front) \draw[draw=black] (0,0) ellipse (2cm and 1cm); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body:** Drawn as an ellipse centered at `(0,0)` with a width of `4cm` and a height of `2cm`. - **Legs and Hooves:** Four legs are represented by lines extending downward, each ending with a small rectangle filled in black to represent hooves. - **Neck and Head:** The neck is a line connecting the body to the head, which is a circle at `(2,2)`. - **Ear and Horn:** The ear is a small triangle above the head. The horn is represented by a filled triangle colored in yellow. - **Eye:** A small filled circle on the head. - **Mane and Tail:** Curved lines drawn with a thicker red pen to represent the mane flowing along the neck and the tail at the back. - **Outline:** The body outline is redrawn at the end to ensure it appears on top of other elements. **Instructions to Compile:** 1. Save the code in a file with a `.tex` extension, for example, `unicorn.tex`. 2. Make sure you have LaTeX installed with the `tikz` package (most LaTeX distributions include it by default). 3. Compile the document using a LaTeX compiler that supports TikZ, such as `pdflatex`: ``` pdflatex unicorn.tex ``` 4. Open the generated PDF to view the unicorn illustration. **Example Output:**  Feel free to customize the colors, sizes, and positions to better suit your needs!
Certainly! Here's a simple TikZ code that draws a unicorn. The drawing uses basic shapes and paths to create a stylized unicorn. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Body \draw[fill=white!90!gray, draw=black] (0,0) ellipse (2cm and 1cm); % Head \draw[fill=white!90!gray, draw=black] (2,1) circle (0.7cm); % Horn \draw[fill=yellow!80!orange, draw=black] (2.5,1.8) -- (2.7,3) -- (2.9,1.8) -- cycle; % Eye \fill (2.3,1.1) circle (0.07cm); % Mane \draw[line width=1pt, red!70!orange] (1.5,1.8) .. controls (1,2.5) and (0.5,2) .. (0.2,1.5) .. controls (0,1) .. (0.5,0.5); % Legs \draw[line width=2pt] (-1.2,-1) -- (-1.2,-2.5) (-0.4,-1) -- (-0.4,-2.5) (0.4,-1) -- (0.4,-2.5) (1.2,-1) -- (1.2,-2.5); % Tail \draw[thick, red!70!orange] (-2,0) .. controls (-3,-0.5) and (-3,-1.5) .. (-2,-2); \end{tikzpicture} \end{document} ``` **Instructions to Compile:** 1. **Save the code**: Copy the code above into a text editor and save it with a `.tex` extension, e.g., `unicorn.tex`. 2. **Compile the code**: Use a LaTeX compiler that supports TikZ (such as `pdflatex`) to compile the file. In a command line, navigate to the directory containing `unicorn.tex` and run: ``` pdflatex unicorn.tex ``` 3. **View the output**: After compilation, a PDF file named `unicorn.pdf` will be generated. Open this file to view the unicorn drawing. **Notes:** - The code uses basic shapes like ellipses and circles to construct the unicorn's body and head. - Bézier curves (`\draw` with `controls`) are used to create the mane and tail with smooth curves. - Feel free to adjust the colors, positions, and shapes to customize the unicorn to your liking. - Ensure you have TikZ installed and included by using `\usepackage{tikz}` and the `[tikz]` option in the `\documentclass`. **Example Output:** The code will generate a simple illustration similar to this:  *(Note: Since we can't display images here, you'll see the output when you compile the code.)* **Troubleshooting:** - If you encounter any errors during compilation, make sure all packages are properly included and that your LaTeX distribution is up to date. - TikZ is a powerful graphics package but can be sensitive to syntax. Double-check for any missing semicolons or braces. Enjoy your unicorn drawing!
Sure! Here's a simple unicorn drawn using TikZ in LaTeX: ```latex \documentclass[border=2mm]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Draw the body \draw[fill=white] (0,0) ellipse (2cm and 1cm); % Draw the neck \draw[fill=white] (1.5,0.5) -- (2.5,2) -- (1.8,2) -- (1,0.8) -- cycle; % Draw the head \draw[fill=white] (2.5,2) circle (0.5cm); % Draw the horn \draw[fill=yellow] (2.7,2.4) -- (3.5,3.5) -- (2.6,2.1) -- cycle; % Draw the eye \fill (2.6,2.1) circle (0.05cm); % Draw the ears \draw[fill=white] (2.3,2.7) -- (2.5,3.2) -- (2.7,2.7) -- cycle; % Draw the legs \draw[fill=white] (-1.2,-1) rectangle (-0.8,-2.5); \draw[fill=white] (-0.5,-1) rectangle (-0.1,-2.5); \draw[fill=white] (0.5,-1) rectangle (0.9,-2.5); \draw[fill=white] (1.2,-1) rectangle (1.6,-2.5); % Draw the tail \draw[thick] (-2,0) .. controls (-2.5,0.5) and (-2.5,-0.5) .. (-2,-1); % Add some mane \draw[thick] (1.2,1.5) .. controls (0.8,2) and (1,2.5) .. (0.5,3); \draw[thick] (1.4,1.3) .. controls (1,1.8) and (1.2,2.3) .. (0.7,2.8); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body:** Drawn as an ellipse centered at (0,0) with width 4cm and height 2cm. - **Neck:** A polygon connecting points to simulate the neck shape. - **Head:** A circle centered at (2.5,2) with a radius of 0.5cm. - **Horn:** A triangle to represent the horn, filled with yellow color. - **Eye:** A small filled circle. - **Ears:** A triangle above the head circle. - **Legs:** Four rectangles positioned to represent legs. - **Tail:** A Bezier curve to simulate the tail. - **Mane:** Curved lines to represent the mane flowing from the neck. **Usage:** To compile this code: 1. Save it in a file with a `.tex` extension. 2. Compile using `pdflatex` or your preferred LaTeX compiler that supports TikZ. **Output:** The code will generate a simple illustration of a unicorn. Here's a preview of what it looks like:  *(Note: As a text-based AI developed by OpenAI, I can't display images directly, but compiling the code will produce the drawing.)* Feel free to customize the drawing by adjusting coordinates, colors, and adding more details!
Certainly! Below is a TikZ code that draws a simplified unicorn using basic shapes and paths. You can compile this code using LaTeX with the TikZ package. The drawing includes the unicorn's body, legs, neck, head, horn, mane, and tail. ```latex \documentclass[tikz, border=10pt]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Body \draw[fill=white, thick] (0,0) ellipse (2cm and 1cm); % Legs \draw[thick] (-1.2,-1) -- (-1.2,-2); \draw[thick] (-0.4,-1) -- (-0.4,-2); \draw[thick] (0.4,-1) -- (0.4,-2); \draw[thick] (1.2,-1) -- (1.2,-2); % Hooves \draw[fill=black] (-1.2,-2) rectangle (-1,-2.2); \draw[fill=black] (-0.4,-2) rectangle (-0.2,-2.2); \draw[fill=black] (0.4,-2) rectangle (0.6,-2.2); \draw[fill=black] (1.2,-2) rectangle (1.4,-2.2); % Neck \draw[fill=white, thick] (1.5,0.5) -- (2.5,2.5) -- (3,2.2) -- (2,0) -- cycle; % Head \draw[fill=white, thick] (2.5,2.5) circle (0.5cm); % Horn \draw[fill=yellow, thick] (2.7,3) -- (2.5,3.8) -- (2.3,3) -- cycle; % Eye \draw[fill=black] (2.6,2.6) circle (0.05cm); % Mane \draw[thick, red] (1.7,1.5) .. controls (1,2.5) and (2,3) .. (2.5,2.5); \draw[thick, red] (2.5,2.5) .. controls (3.5,2.5) and (3,1.5) .. (2.7,1.8); % Tail \draw[thick, red] (-2,0) .. controls (-2.5,0.5) and (-2.5,-0.5) .. (-2,-1); \end{tikzpicture} \end{document} ``` **Explanation:** - **Body:** An ellipse is drawn to represent the unicorn's body. - **Legs:** Four lines are drawn downward from the body to represent the legs. - **Hooves:** Small rectangles at the end of each leg to depict hooves, filled in black. - **Neck:** A polygon connecting points to form the neck shape. - **Head:** A circle represents the head of the unicorn. - **Horn:** A triangle filled with yellow color to represent the horn on the head. - **Eye:** A small black circle on the head. - **Mane:** Curved lines with red color to depict the mane flowing from the neck and head. - **Tail:** A curved line at the back of the body to represent the tail. **Instructions:** 1. Save the above code in a file with a `.tex` extension, for example, `unicorn.tex`. 2. Compile the file using LaTeX with the `tikz` package installed. You can use `pdflatex` for compilation: ``` pdflatex unicorn.tex ``` 3. Open the generated PDF to view the unicorn drawing. **Note:** - This is a simplified representation of a unicorn using basic TikZ commands. - Feel free to adjust the coordinates, colors, and styles to customize the unicorn to your liking. - Ensure that you have a LaTeX distribution installed that includes the TikZ package. **Example Output:** The compiled code will produce a drawing similar to this (conceptual representation):  If you need further assistance or want to add more details to the unicorn, please let me know!
Certainly! You can draw a unicorn in LaTeX using the `tikzlings` package, which provides a collection of cute animals including a unicorn. Here's how you can do it: 1. **Install the `tikzlings` package**: Make sure you have the latest version of the `tikzlings` package. You can download it from [CTAN](https://ctan.org/pkg/tikzlings) or install it through your LaTeX package manager. 2. **Use the following code in your LaTeX document**: ```latex \documentclass{article} \usepackage{tikzlings} \begin{document} \begin{tikzpicture} \unicorn[body=white!90!black, mane=purple!80!blue, tail=purple!80!blue] \end{tikzpicture} \end{document} ``` **Explanation of the code:** - The `\usepackage{tikzlings}` line includes the `tikzlings` package. - Within the `tikzpicture` environment, the `\unicorn` command draws a unicorn. - The optional parameters `[body=..., mane=..., tail=...]` allow you to customize the colors: - `body`: Color of the unicorn's body. - `mane`: Color of the mane and hooves. - `tail`: Color of the tail. - You can adjust the colors using standard LaTeX color definitions. **Example Output:**  *(Note: Replace with actual output if possible; since images can't be embedded here, you'll need to compile the code to see the unicorn.)* **Additional Customization:** You can further customize the unicorn by adding more options: - `unihorn`: Color of the horn. - `eye`: Color of the eye. - `scale`: Size of the unicorn. **Example with More Customization:** ```latex \documentclass{article} \usepackage{tikzlings} \begin{document} \begin{tikzpicture} \unicorn[ body=white, mane=rainbow, tail=rainbow, unihorn=gold, eye=black, scale=2 ] \end{tikzpicture} \end{document} ``` In this example: - The unicorn's mane and tail are set to `rainbow`. - The horn (`unihorn`) is colored `gold`. - The size is doubled with `scale=2`. **Compiling the Document:** To compile the LaTeX document and see the unicorn: 1. Save the code in a file with a `.tex` extension. 2. Compile it using a LaTeX editor like TeXstudio, Overleaf, or via command line with `pdflatex`. 3. Ensure all packages are updated to the latest versions. **Notes:** - The `tikzlings` package depends on `tikz` and other TikZ libraries. These are usually included in most LaTeX distributions. - If you encounter any errors, make sure all packages are properly installed and updated. **References:** - [tikzlings Package Documentation](https://ctan.org/pkg/tikzlings) - [CTAN TikZ and PGF](https://ctan.org/pkg/pgf) Feel free to adjust the colors and parameters to create your perfect unicorn!
Certainly! Here's a TikZ code snippet that draws a simple unicorn. You can compile this code using LaTeX to see the unicorn drawing. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \begin{document} \begin{tikzpicture} % Set line and fill colors \tikzstyle{unicornbody}=[draw=black, fill=white] \tikzstyle{unicornmane}=[draw=black, fill=purple!70!blue] \tikzstyle{unicornhorn}=[draw=black, fill=yellow] \tikzstyle{unicorneye}=[draw=black, fill=black] % Body \draw[unicornbody] (0,0) ellipse (1.5cm and 1cm); % Legs \foreach \x in {-0.8, -0.4, 0.4, 0.8} { \draw[unicornbody] (\x,-1) -- (\x+0.1,-1.8) -- (\x+0.3,-1.8) -- (\x+0.2,-1) -- cycle; } % Neck \draw[unicornbody] (1,0.5) -- (1.5,1.5) -- (1,2.5) -- (0.5,1.5) -- cycle; % Head \draw[unicornbody] (1,2.5) circle (0.5cm); % Horn \draw[unicornhorn] (1,3) -- (1.1,3.8) -- (0.9,3.8) -- cycle; % Mane \draw[unicornmane] (0.6,2.8) .. controls (0.2,3.5) and (0.2,1.5) .. (0.6,2.2) -- (0.6,2.8); % Tail \draw[unicornmane] (-1.5,0.5) .. controls (-2,1) and (-2,-0.5) .. (-1.5,-1); % Eye \draw[unicorneye] (1.1,2.6) circle (0.05cm); % Ground \draw[black!50] (-2,-1.8) -- (2,-1.8); \end{tikzpicture} \end{document} ``` **Instructions to Compile the Code:** 1. **Save the Code:** Save the code snippet above in a file named `unicorn.tex`. 2. **Compile the Document:** Run the following command in your terminal to compile the LaTeX document: ```bash pdflatex unicorn.tex ``` 3. **View the Output:** Open the generated `unicorn.pdf` file to view the drawing. **Explanation of the Code:** - **Styles Defined:** - `unicornbody`: Style for the unicorn's body parts (white fill with black outline). - `unicornmane`: Style for the mane and tail (purple-blue fill). - `unicornhorn`: Style for the horn (yellow fill). - `unicorneye`: Style for the eye (black fill). - **Shapes Used:** - **Ellipses and Circles:** Used for the body and head. - **Polygons (`\draw ... -- ... -- cycle;`):** Used for legs, neck, and horn. - **Curved Lines (`\draw ... .. controls ... .. ...;`):** Used for mane and tail. - **Foreach Loop (`\foreach`):** Draws the four legs at specified positions. - **Drawing Order:** - The body is drawn first, followed by the legs, neck, head, horn, mane, tail, and eye to layer the components correctly. **Customization:** - **Colors:** - You can change the colors in the `\tikzstyle` definitions to customize the unicorn's appearance. - **Mane and Tail Shape:** - Adjust the control points in the `\draw[unicornmane]` commands to change the mane and tail flow. - **Size Adjustments:** - Modify the dimensions in the shapes (e.g., ellipse radii, circle radius) to resize different parts. **Note:** - This is a simplified representation of a unicorn. TikZ allows for highly detailed drawings, so feel free to enhance the illustration by adding more features or refining existing ones. - If you're new to TikZ, experimenting with the code and consulting the TikZ manual can help you learn more about drawing complex shapes. **Example Output:** The compiled code will produce a drawing similar to this:  *(Note: As this is a text-based platform, the image is a placeholder to give you an idea of what the output looks like after compilation.)*
Certainly! Below is a TikZ code that draws a simplified unicorn using basic shapes and paths. You can compile this code using LaTeX with the TikZ package. ```latex \documentclass[tikz]{standalone} \usepackage{tikz} \usetikzlibrary{decorations.pathmorphing} \begin{document} \begin{tikzpicture}[scale=2, thick] % Body \draw[fill=white] (0,0) ellipse (1cm and 0.5cm); % Neck \draw[fill=white] (0.6,0.3) -- (0.9,1) -- (0.5,1) -- (0.3,0.4) -- cycle; % Head \draw[fill=white] (0.9,1) .. controls (1.2,1.3) and (1.0,1.5) .. (0.7,1.4) -- (0.5,1) -- cycle; % Horn \draw[fill=yellow] (0.85,1.5) -- (0.95,1.9) -- (0.75,1.6) -- cycle; % Ear \draw[fill=white] (0.78,1.45) -- (0.85,1.6) -- (0.75,1.5) -- cycle; % Eye \fill (0.82,1.25) circle (0.02cm); % Legs \draw (-0.5,-0.5) -- (-0.5,-1); \draw (-0.2,-0.5) -- (-0.2,-1); \draw (0.2,-0.5) -- (0.2,-1); \draw (0.5,-0.5) -- (0.5,-1); % Tail \draw[decorate, decoration={coil, amplitude=2pt, segment length=5pt}] (-1,0) .. controls (-1.2,0.2) and (-1.2,-0.2) .. (-1,-0.1); % Mane \draw[decorate, decoration={coil, amplitude=2pt, segment length=3pt}] (0.7,1.4) .. controls (0.4,1.6) and (0.3,1.2) .. (0.4,1); % Outline (optional) % \draw (0,0) ellipse (1cm and 0.5cm); % \draw (0.6,0.3) -- (0.9,1) -- (0.5,1) -- (0.3,0.4) -- cycle; % \draw (0.9,1) .. controls (1.2,1.3) and (1.0,1.5) .. (0.7,1.4) -- (0.5,1) -- cycle; \end{tikzpicture} \end{document} ``` **Explanation of the Code:** - **Preamble:** - We use the `standalone` document class for compiling just the TikZ picture. - The `tikz` package is included for drawing capabilities. - The `decorations.pathmorphing` library is included to create decorative paths like the mane and tail. - **Drawing the Unicorn:** - **Body:** An ellipse centered at `(0,0)` with a horizontal radius of `1cm` and vertical radius of `0.5cm`. ```latex \draw[fill=white] (0,0) ellipse (1cm and 0.5cm); ``` - **Neck:** A polygon connecting points to form the neck. ```latex \draw[fill=white] (0.6,0.3) -- (0.9,1) -- (0.5,1) -- (0.3,0.4) -- cycle; ``` - **Head:** A closed path using Bézier curves to form the head. ```latex \draw[fill=white] (0.9,1) .. controls (1.2,1.3) and (1.0,1.5) .. (0.7,1.4) -- (0.5,1) -- cycle; ``` - **Horn:** A triangle filled with yellow to represent the horn. ```latex \draw[fill=yellow] (0.85,1.5) -- (0.95,1.9) -- (0.75,1.6) -- cycle; ``` - **Ear:** A small triangle for the ear. ```latex \draw[fill=white] (0.78,1.45) -- (0.85,1.6) -- (0.75,1.5) -- cycle; ``` - **Eye:** A small filled circle. ```latex \fill (0.82,1.25) circle (0.02cm); ``` - **Legs:** Four lines extending downward from the body. ```latex \draw (-0.5,-0.5) -- (-0.5,-1); \draw (-0.2,-0.5) -- (-0.2,-1); \draw (0.2,-0.5) -- (0.2,-1); \draw (0.5,-0.5) -- (0.5,-1); ``` - **Tail:** A decorative path representing the tail. ```latex \draw[decorate, decoration={coil, amplitude=2pt, segment length=5pt}] (-1,0) .. controls (-1.2,0.2) and (-1.2,-0.2) .. (-1,-0.1); ``` - **Mane:** Another decorative path for the mane. ```latex \draw[decorate, decoration={coil, amplitude=2pt, segment length=3pt}] (0.7,1.4) .. controls (0.4,1.6) and (0.3,1.2) .. (0.4,1); ``` - **Outline (Optional):** Commented out lines that you can enable to see the outlines of the shapes. **How to Compile the Code:** 1. **Save the Code:** - Copy the code and save it in a file named, for example, `unicorn.tex`. 2. **Compile with LaTeX:** - Open a terminal or command prompt. - Navigate to the directory containing `unicorn.tex`. - Run the following command to compile the document: ``` pdflatex unicorn.tex ``` - This will generate a PDF file named `unicorn.pdf` containing the drawing. 3. **View the Output:** - Open `unicorn.pdf` with a PDF viewer to see the unicorn drawing. **Adjusting the Drawing:** - **Scaling:** - Modify the `scale` parameter in the `\begin{tikzpicture}` line to make the drawing larger or smaller. ```latex \begin{tikzpicture}[scale=2, thick] ``` - **Colors:** - Change the colors by modifying the `fill` and `draw` options in the `\draw` commands. - **Shapes and Positions:** - Adjust the coordinates in the drawing commands to change the shapes and positions of the unicorn's features. **Additional Notes:** - The unicorn is drawn using simple geometric shapes to keep the code manageable. - The `decorate` option with `coil` decoration is used to create a stylized mane and tail. - Feel free to customize the unicorn by adding more details or styling elements as desired. **Example Output:** *Here's a rough illustration of what the unicorn will look like:*  *(Note: Replace the image link with an actual compiled version if possible.)* --- I hope this helps you create the unicorn drawing you need! If you have any questions or need further assistance with customizing the drawing, feel free to ask.